vue3.js + vite + Typescript,使用过程中趟过的坑
defineModel() is a compiler-hint helper that is only usable inside <script setup> of a single file component. Its arguments should be compiled away and passing it at runtime has no effect.
defineModel()是一个编译器提示帮助器,只能在脚本setup单个文件组件的。它的参数应该被编译掉,并且在运行时传递它没有影响。
// 父组件
<script lang="ts" setup>
import { ref } from 'vue'
import CustomInput from './CustomInput.vue'
const inputValue = ref('hello defineModel')
</script>
<template>
<div>
<CustomInput v-model="inputValue"></CustomInput>
<p>Input value: {{ inputValue }}</p>
</div>
</template>
// CustomInput.vue
<script setup lang="ts">
import { defineModel } from 'vue'
const inputVal = defineModel()
</script>
<template>
<div class="custom-input">
<input v-model="inputVal" type="text" />
</div>
</template>
package.json 项目依赖还是会报错, 不能正常使用。
“vue”: “^3.3.4”,
defineModel 宏默认是关闭的,使用它需要手动开启。vite.config.ts配置如下:
export default defineConfig({
plugins: [
vue({
script: {
// 开启 defineModel
defineModel: true
}
})
]
})
找不到模块“@/views/elements.vue”或其相应的类型声明。

报错原因:typescript 只能理解 .ts 文件,无法理解 .vue文件
解决方法:
1、在项目根目录或 src 文件夹下创建一个后缀为 .d.ts 的文件,并写入以下内容:
declare module '*.vue' {
import { ComponentOptions } from 'vue'
const componentOptions: ComponentOptions
export default componentOptions
}
2、也可执行下面的命令
npm i –save-dev @types/node
Vite alias 类型别名配置
// vite.config.ts
export default defineConfig({
resolve: {
alias: {
"@": path.join(__dirname, "./src")
},
}
})
使用 TypeScript 时,编辑器会提示路径不存在的警告⚠️
解决可以在 tsconfig.json
中添加 compilerOptions.paths
的配置:
{
"compilerOptions": {
"paths": {
"@/*": ["./src/*"]
}
}
}
vite
打包报错/告警
element-plus 的 index.css 文件包含 @chartset:UTF-8
“@charset” must be the first rule in the file }@charset “UTF-8”;
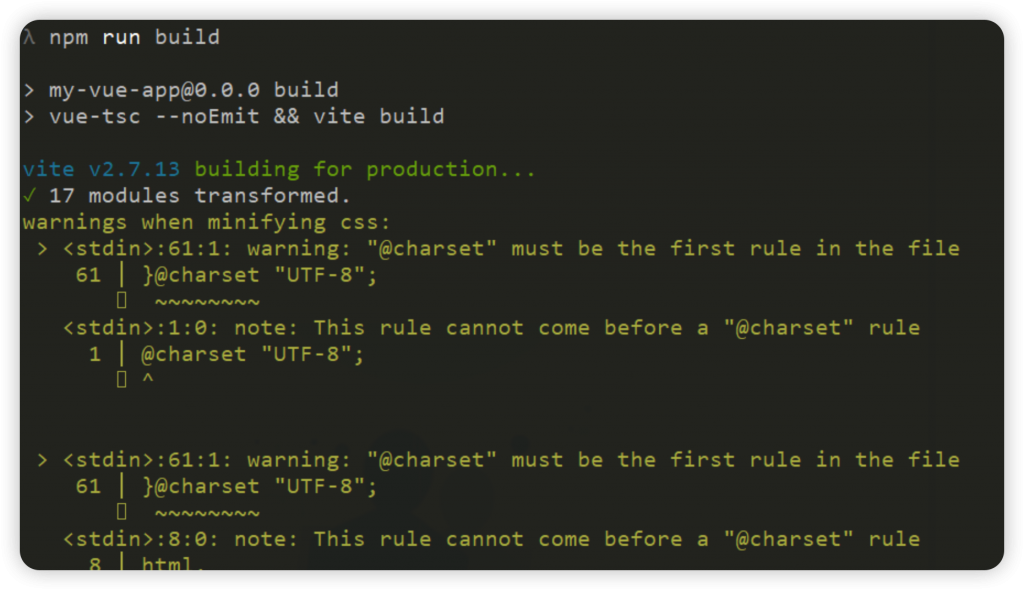
在vite.config.js里面,加一个sass / less 的配置,把 charset 关掉就行了
export default defineConfig({
css: {
preprocessorOptions: {
scss: {
charset: false
}
}
}
})